Technical stack
Technologies at play in Onyxia-web
To find your way in Onyxia, the best approach is to start by getting a surface-level understanding of the libraries that are leveraged in the project.
tsafe 🐔
We also heavily rely on tsafe. It's a collection of utilities that help write cleaner TypeScript code. It is crutial to understand at least assert
, id, Equals and symToStr to be able to contribute on the codebase.
For working on what the end user 👁
Anything contained in the src/ui directory.
Onyxia-UI 🐔
The UI toolkit used in the project, you can find the setup of onyxia-UI in onyxia-web here: src/ui/theme.tsx.
MUI integration
Onyxia-UI is fully compatible with MUI.
Onyxia-UI offers a library of reusable components but you can also use MUI components in the project, their aspect will automatically be adapted to blend in with the theme.
🔡 Linking onyxia-ui in onyxia-web
To release a new version of Onyxia-UI. You just need to bump the package.json's version and push. The CI will automate publish a new version on NPM.
If you want to test some changes made to onyxia-ui in onyxia-web before releasing a new version of onyxia-ui to NPM you can link locally onyxia-ui in onyxia-web.
cd ~/github
git clone https//github.com/InseeFrLab/onyxia
cd onyxia/web
yarn install
cd ~/github/onyxia #This is just a suggestion, clone wherever you see fit.
git clone https://github.com/InseeFrLab/onyxia-ui ui
cd ui
yarn install
yarn build
yarn link-in-web
npx tsc -w
# Open a new terminal
cd ~/github/onyxia/web
yarn start
Now you can make changes in ~/github/onyxia/ui/
and see the live updates.
If you want to install/update some dependencies, you must remove the node_modules, do you updates, then link again.
tss-react 🐔
The library we use for styling.
Rules of thumbs when it comes to styling:
Every component should accept an optional
className
prop it should always overwrite the internal styles.A component should not size or position itself. It should always be the responsibility of the parent component to do it. In other words, you should never have
height
,width
,top
,left
,right
,bottom
ormargin
in the root styles of your components.You should never have a color or a dimension hardcoded elsewhere than in the theme configuration. Use
theme.spacing()
(ex1, ex2, ex3) andtheme.colors.useCases.xxx
.
screen-scaler 🐔
Onyxia is mostly used on desktop computer screens. It's not worth the effort to create a fully flege responsive design for the UI. screen-scaler enables us to design for a sigle canonical screen size. The library take charge of scaling/shrinking the image. depending on the real size of the screen. It also asks to rotate the screen when the app is rendered in protrait mode.
Storybook
It enables us to test the graphical components in isolation. See sources.
To launch Storybook locally run the following command:
yarn storybook
vite-envs 🐔
We need to be able to do:
docker run --env OIDC_URL="https://url-of-our-keycloak.fr/auth" InseeFrLab/onyxia-web
Then, somehow, access OIDC_URL
in the code like process.env["OIDC_URL"]
.
In theory it shouldn't be possible, onyxia-web is an SPA, it is just static JS/CSS/HTML. If we want to bundle values in the code, we should have to recompile. But this is where cra-envs
comes into play.
It enables to run onyxia-web again a specific infrastructure while keeping the app docker image generic.
Checkout the helm chart:
web:
replicaCount: 2
env:
MINIO_URL: https://minio.lab.sspcloud.fr
VAULT_URL: https://vault.lab.sspcloud.fr
OIDC_URL: https://auth.lab.sspcloud.fr/auth
OIDC_REALM: sspcloud
TITLE: SSP Cloud
All the accepted environment variables are defined here: .env. They are all prefixed with
REACT_APP_
to be compatible with create-react-app. Default values are defined in this file.Only in development (
yarn start
).env.local
is also loaded and have priority over.env
Then, in the code the variable can be accessed like this.
powerhooks 🐔
It's a collection general purpose react hooks. Let's document the few use cases you absolutely need to understand:
Avoiding useless re-render of Components
For the sake of performance we enforce that every component be wrapped into React.memo()
. It makes that a component only re-render if one of their prop has changed.
However if you use inline functions or useCallback
as callbacks props your components will re-render every time anyway:
We always use useConstCallback for callback props. And useCallbackFactory
for callback prop in lists.
Measuring Components
It is very handy to be able to get the height and the width of components dynamically. It prevents from having to hardcode dimension when we don’t need to. For that we use useDomRect
``
Keycloakify 🐔
It's a build tool that enables to implement the login and register pages that users see when they are redirected to Keycloak for authentication.
If the app is being run on Keycloak the kcContext
isn't undefined
and it means shat we should render the login/register pages.
If you want to test, uncomment this line and run yarn start
. You can also test the login pages in a local keycloak container by running yarn keycloak
. All the instructions will be printed on the console.
The keycloak-theme.jar
file is automatically build and uploaded as a GitHub release asset by the CI.
type-routes
The library we use for routing. It's like react-router but type safe.
i18nifty 🐔
For internalization and translation.
Vite
For working on 🧠 of the App
Anything contained in the src/core directory.
clean-architecture 🐔
The framework used to implement strict separation of concern betwen the UI and the Core and high modularity of the code.
There is a snake game (the classic nokia game) example for helping you understand the clean architecture framework.
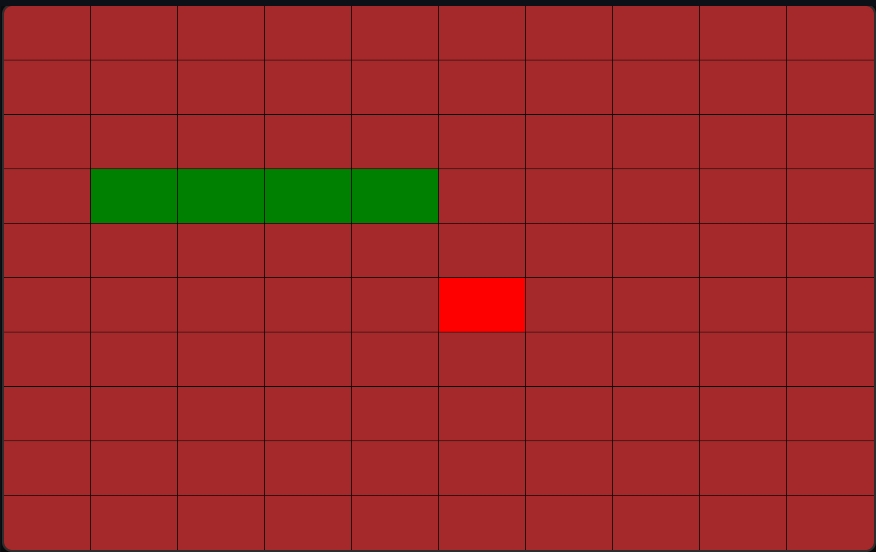
oidc-spa 🐔
For everything related to user authentication.
EVT 🐔
EVT is an event management library (like RxJS is).
A lot of the things we do is powered under the hood by EVT. You don't need to know EVT to work on onyxia-web however, in order to demystify the parts of the codes that involve it, here are the key ideas to take away:
If we need to perform particular actions when a value gets changed, we use
StatefullEvt
.We use
Ctx
to detaches event handlers when we no longer need them. (See line 108 on this playground)In React, we use the useEvt hook to work with DOM events.
Was this helpful?